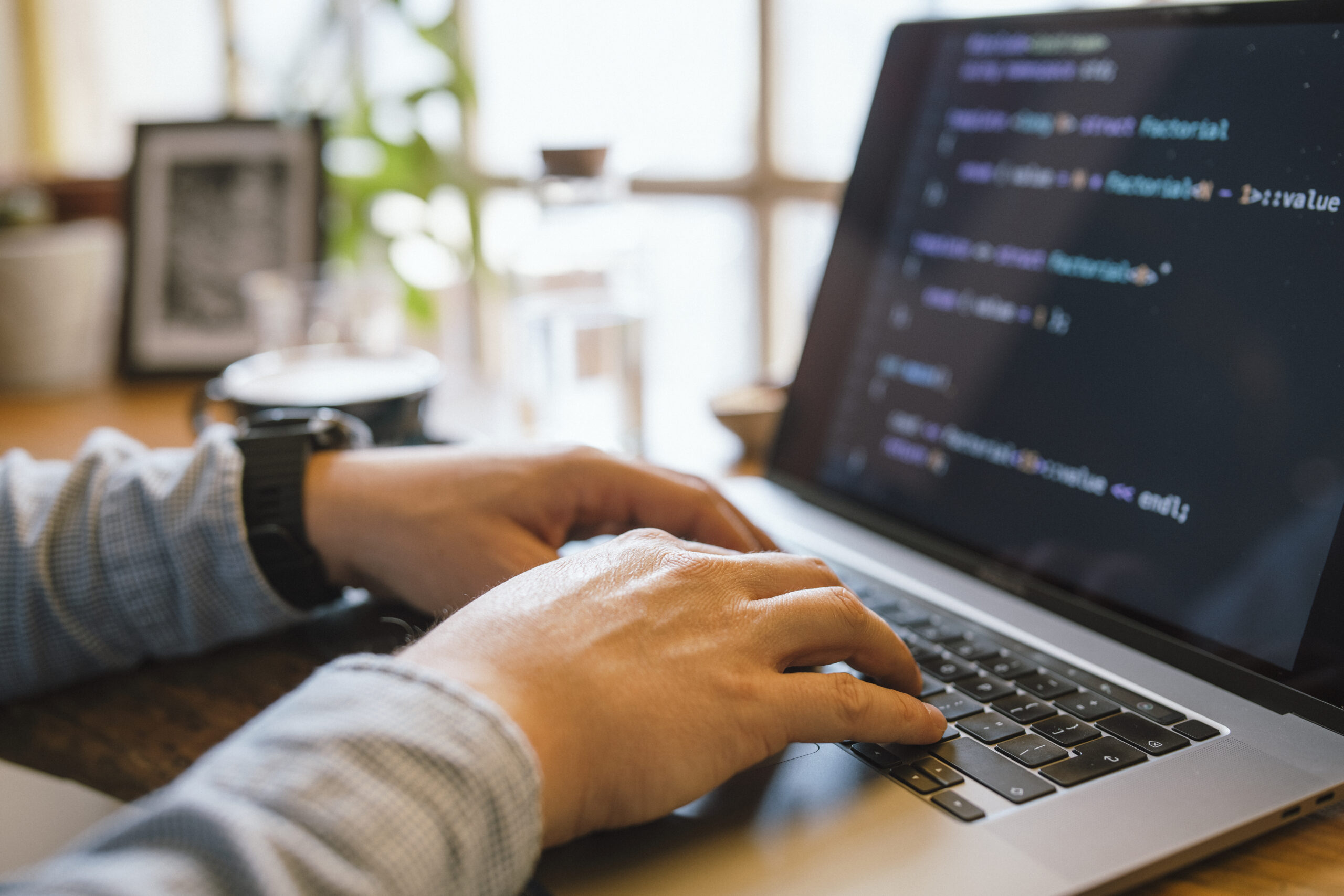
Debugging is one of the most important — nevertheless normally overlooked — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go wrong, and Studying to Feel methodically to solve difficulties successfully. Whether you are a starter or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and considerably transform your productiveness. Allow me to share many approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging techniques is by mastering the instruments they use daily. Whilst creating code is 1 part of progress, figuring out the best way to interact with it correctly for the duration of execution is equally important. Modern-day advancement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these equipment can do.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources let you established breakpoints, inspect the value of variables at runtime, stage through code line by line, and perhaps modify code about the fly. When utilized the right way, they Enable you to notice particularly how your code behaves in the course of execution, which is priceless for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-stop builders. They let you inspect the DOM, keep track of community requests, view authentic-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can turn annoying UI issues into manageable tasks.
For backend or system-degree builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of running processes and memory management. Mastering these tools could have a steeper Mastering curve but pays off when debugging overall performance concerns, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Command systems like Git to know code historical past, come across the precise instant bugs were being introduced, and isolate problematic modifications.
Eventually, mastering your equipment suggests likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your advancement setting to ensure when difficulties occur, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out fixing the particular problem instead of fumbling through the process.
Reproduce the Problem
One of the most critical — and infrequently missed — ways in productive debugging is reproducing the situation. Ahead of jumping in to the code or making guesses, builders will need to make a steady atmosphere or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a video game of possibility, usually leading to squandered time and fragile code alterations.
The first step in reproducing a problem is collecting as much context as feasible. Question queries like: What steps brought about the issue? Which natural environment was it in — growth, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
After you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood natural environment. This could signify inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the sting circumstances or point out transitions involved. These exams don't just assist expose the situation but also avert regressions Down the road.
Occasionally, The problem may very well be surroundings-precise — it'd occur only on specified running units, browsers, or under certain configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It requires patience, observation, and also a methodical solution. But once you can constantly recreate the bug, you are previously midway to repairing it. That has a reproducible state of affairs, You may use your debugging tools more successfully, check prospective fixes securely, and talk a lot more Obviously using your staff or people. It turns an summary grievance into a concrete problem — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Incorrect. Instead of seeing them as disheartening interruptions, builders need to understand to treat mistake messages as immediate communications through the technique. They usually tell you what precisely took place, in which it happened, and in some cases even why it took place — if you understand how to interpret them.
Start by examining the concept very carefully As well as in whole. Several developers, particularly when below time tension, look at the very first line and straight away start out producing assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste mistake messages into search engines like google and yahoo — read through and understand them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some problems are imprecise or generic, and in Individuals scenarios, it’s essential to examine the context through which the mistake happened. Verify relevant log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger issues and provide hints about prospective bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an application behaves, supporting you fully grasp what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging stages contain DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for comprehensive diagnostic information during development, Facts for normal gatherings (like profitable commence-ups), WARN for opportunity challenges that don’t split the appliance, ERROR for precise challenges, and Deadly when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. Excessive logging can obscure crucial messages and slow down your process. Target important events, condition modifications, enter/output values, and significant choice details within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. By using a well-imagined-out logging solution, you are able to decrease the time it's going to take to spot troubles, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex task—it's a kind of investigation. To proficiently identify and repair bugs, developers ought to solution the process like a detective solving a thriller. This frame of mind allows stop working complex concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs of the challenge: mistake messages, incorrect output, or functionality difficulties. Identical to a detective surveys against the law scene, obtain just as much applicable information and facts as you can without leaping to conclusions. Use logs, exam cases, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What could possibly be creating this behavior? Have any changes just lately been designed on the codebase? Has this situation occurred before under identical situation? The aim is always to narrow down alternatives and establish likely culprits.
Then, check your theories systematically. Try to recreate the problem in a very controlled atmosphere. If you suspect a certain operate or component, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code queries and Enable the final results lead you nearer to the truth.
Pay near interest to compact information. Bugs frequently disguise inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular error, or maybe a race problem. Be complete and individual, resisting the urge to patch The difficulty with no fully comprehension it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging process can preserve time for upcoming concerns and enable Other people recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden concerns in advanced units.
Create Exams
Producing checks is among the simplest methods to increase your debugging techniques and In general improvement efficiency. Exams not merely enable capture bugs early but will also function a security net that gives you self-confidence when producing alterations on your codebase. A very well-analyzed software is much easier to debug as it helps you to pinpoint accurately where and when a problem occurs.
Get started with device checks, which deal with unique capabilities or modules. These smaller, isolated checks can promptly expose no matter if a certain bit of logic is Functioning as anticipated. Whenever a test fails, you immediately know where to look, noticeably lessening time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear following previously remaining fastened.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These help make sure several areas of your application do the job collectively easily. They’re particularly handy for catching bugs that take place in complex devices with several components or expert services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to think critically regarding your code. To check a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing naturally leads to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails regularly, it is possible to focus on fixing the bug and enjoy your test pass when The problem is fixed. This method makes sure that the exact same bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing match right into a structured and predictable process—aiding you catch additional bugs, faster and much more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your head, cut down annoyance, and sometimes see The problem from a new viewpoint.
When you are also near to the code for also extended, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a coffee break, or even switching to another endeavor for ten–15 minutes can refresh your concentrate. Many builders report obtaining the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly all through extended debugging periods. Sitting before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You could out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
In short, using breaks is not really a sign of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each one can teach you one thing worthwhile for those who take the time to reflect and evaluate what went Improper.
Start off by inquiring on your own a handful of key questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with far better procedures like unit screening, code evaluations, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be a wonderful practice. Retain a developer journal or retain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. After some time, you’ll begin to see patterns—recurring problems or common issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your peers can be In particular strong. Regardless of whether it’s through a Slack information, a short generate-up, or A fast understanding-sharing session, helping Some others stay away from the same challenge boosts group performance and cultivates a more robust Studying tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers are certainly not the ones who publish perfect code, but people that continuously understand from their mistakes.
In the long run, each bug you correct provides a fresh layer on your skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, remember: debugging isn’t a chore — it’s read more a chance to become superior at Anything you do.